8. Operators
In general, operator code blocks process inputs (they do something with the inputs) and returns an output.
8.1. Math
Math operators do the typical math things.
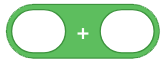
This code block adds two numbers.
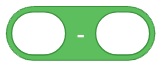
This code block subtracts two numbers.
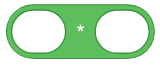
This code block multiplies two numbers.
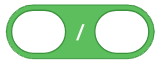
This code block divides two numbers.
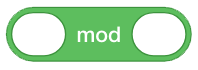
This code block returns the remainder of two numbers.
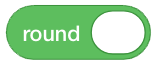
This code block rounds a number.
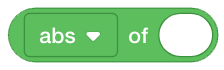
This code block returns the absolute value of a number.
8.2. Random numbers
You may also generate random numbers.
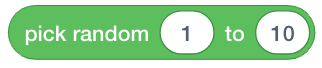
This code block is used to generate random numbers.
8.3. Comparison
Comparison code blocks returns either True
or False
.
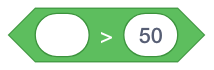
This code block compares if the left value is greater than the right value.
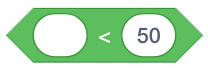
This code block compares if the left value is less than the right value.
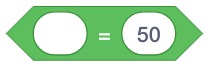
This code block compares if the left and right values are equal.
8.4. Boolean operators
You may chain together multiple comparisons.
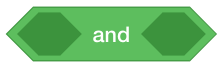
This code block returns True
if both comparisons are True
.
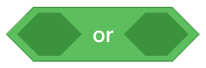
This code block returns True
if one of the comparisons is True
.
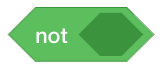
This code block returns True
if the comparison is False
.
8.5. String operations
These are code blocks that may be used to manipulate string values.
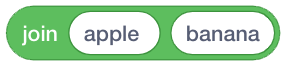
This code block joins (or concatenates
) two strings.
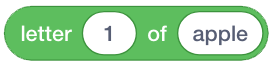
This code block returns the letter at the specified index from the string.
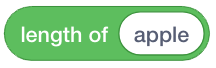
This code block returns the length of the string.
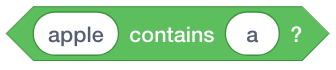
This code block checks to see if the string contains the specified value (or substring
).